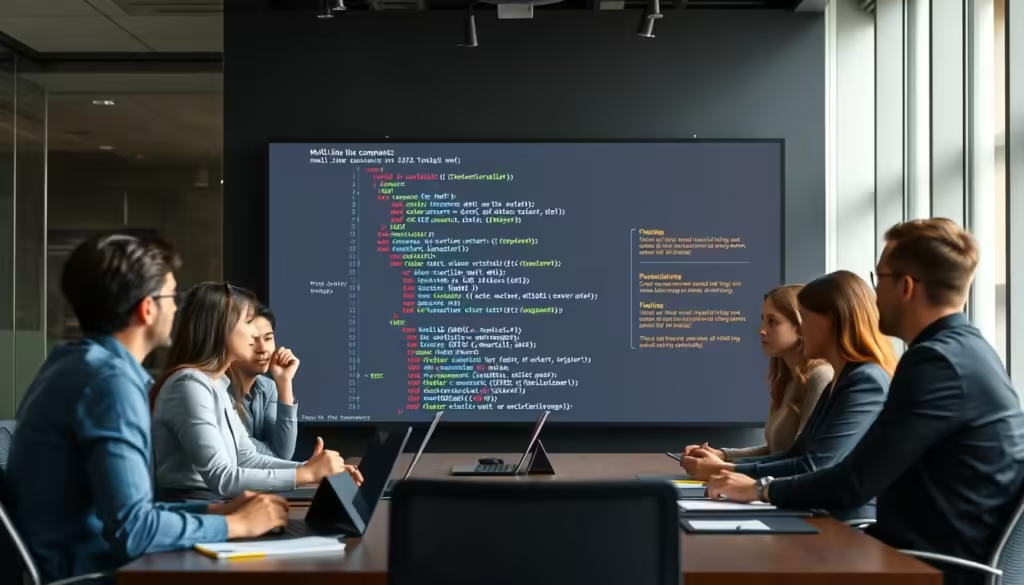
As a software developer, I’ve faced the challenge of keeping code clean and well-documented. It’s not just about writing efficient code or optimizing performance. It’s also about sharing our thought process with future developers. The humble comment plays a crucial role in this, transforming confusing code into a masterpiece.(How to Comment Out Multiple Lines in Python)
In Python, commenting out multiple lines is key. It helps us temporarily disable code, provide explanations, or test different approaches. Python’s lack of a built-in multi-line comment syntax can be frustrating. But, we have tricks to make commenting easy.
Key Takeaways
- Python lacks a built-in mechanism for multi-line comments, requiring workarounds.
- Single-line comments in Python use the hash (#) symbol, while multi-line comments leverage docstrings or consecutive single-line comments.
- Docstrings, enclosed in triple quotes (“”” or ”’), are a versatile solution for multi-line comments in Python.
- Proper formatting and indentation are crucial when using docstrings for commenting purposes.
- Commenting code is essential for code documentation, collaboration, and maintaining code readability over time.
Understanding Comments in Python (How to Comment Out Multiple Lines in Python)
In Python programming, comments are vital for code clarity and upkeep. They are texts ignored by the interpreter, used to add notes and descriptions to code. This doesn’t alter its functionality but enriches its readability.
What are Comments?
Comments in Python serve as a means for developers to share their code’s purpose and logic. They offer insights into complex code, aiding in debugging and project collaboration. This makes them indispensable for understanding and maintaining code.
Importance of Commenting Code
Commenting Python code is crucial for several reasons. It boosts Python code readability by detailing each code block’s purpose. This makes Python code maintenance simpler, as updates become more straightforward.
Comments are also key for teamwork. They help other programmers grasp the code’s logic and intent. This is vital in large projects or when team members change.
Benefits of Commenting Python Code | Examples |
---|---|
Enhance code readability | Explain the purpose of complex algorithms or data structures |
Facilitate code maintenance | Describe the expected input and output of a function |
Support collaboration and knowledge sharing | Provide context for why a particular approach was chosen |
Aid in debugging and troubleshooting | Document known issues or limitations with the current implementation |
“Well-commented code is its own best documentation. As you’re about to add a comment, ask yourself, ‘How can I improve the code so that this comment isn’t needed?'” – Steve McConnell, author of “Code Complete”
By using clear, concise comments, Python developers can significantly improve their code’s quality and longevity. This makes commenting a fundamental practice for any successful software project.
Single-Line vs. Multi-Line Comments
In Python, developers have two main commenting options: single-line and multi-line comments. The choice depends on the context and the information to be shared. Each has its own use case.
When to Use Single-Line Comments (How to Comment Out Multiple Lines in Python)
Single-line comments in Python are marked with the #
symbol. They are perfect for short explanations, code separation, or variable documentation. Following Python conventions, they highlight the most critical parts of a program. Excessive use can, however, decrease readability.
When to Use Multi-Line Comments
For detailed explanations or to disable large code blocks, multi-line comments are preferred. They use triple quotes """
and can span multiple lines. They are often used for function docstrings, module documentation, or complex logic context.
The Python community has differing opinions on multi-line comments. Some prefer consecutive single-line comments over multi-line strings. Yet, Guido van Rossum, Python’s creator, supports multi-line strings for commenting.
The decision between single-line and multi-line comments in Python varies by project needs and developer preference. The goal is to use comments wisely, adding value for readers, whether team members or future developers.
“Code is read much more often than it is written.” – Guido van Rossum, Creator of Python
Understanding when to use single-line and multi-line comments improves code readability, maintainability, and self-documentation. This aligns with Python commenting best practices and Python coding conventions.
Using the Hash Symbol for Multi-Line Comments
In Python, the hash symbol (#) is mainly used for single-line comments. However, it can be challenging when commenting out extensive code blocks. To achieve multi-line comments with the hash symbol, each line must start with the # character.
Syntax for Single-Line Comments
The syntax for a single-line comment in Python is straightforward. Simply place the hash symbol (#) at the line’s start. This instructs the Python interpreter to disregard the line as code, treating it as a comment. For instance:
# This is a single-line comment in Python
Limitations of the Hash Symbol
- To create multi-line comments with the hash symbol, you must add the # character at the start of each line. This can be tedious for extensive code segments.
- The hash symbol is ideal for temporarily commenting out small code sections or adding brief explanations to specific lines.
- For longer, more detailed comments or to enhance code readability, other methods, such as multi-line strings, might be more effective.
While the hash symbol is versatile for commenting in Python, it has limitations for multi-line comments. Recognizing these constraints aids in selecting the best commenting approach for your Python code organization and Python comment syntax requirements.
Multi-Line String as Comment
In Python, multi-line strings, or triple quotes (”’ or “””), serve as comments. This method is great for commenting out large blocks of code. You don’t need to add a comment symbol to each line.
How to Use Triple Quotes
To create a multi-line comment, just wrap your text in triple single quotes (”’) or triple double quotes (“””). This way, your text can cover multiple lines without extra comment symbols. For instance:
”’
This is a multi-line
comment in Python.
It can span
multiple lines.
”’
Advantages of Using Triple Quotes
- Quickly comment out or uncomment large sections of code with ease.
- Ideal for providing detailed documentation or explanations within your code, known as docstrings.
- Maintain readability and clean code by avoiding the need to add a comment symbol to each line.
- Widely used and accepted practice in the Python community, as evidenced by PEP 257 (the Python style guide).
Python doesn’t have a specific syntax for multi-line comments. Yet, triple quotes are a common and effective solution. This technique boosts code organization, readability, and documentation. It’s a key tool for Python developers.
Best Practices for Commenting
Effective commenting is key to keeping Python code readable and maintainable. Following best practices boosts code readability and aids in developer collaboration. Let’s delve into the essential principles for making your Python comments relevant and concise.
Keeping Comments Relevant
As your codebase grows, it’s vital to keep comments current and relevant. Regularly check and update comments with code changes. Outdated or misleading comments can confuse and mislead other developers.
Using Clear and Concise Language
Strive for clarity and brevity in your comments. Steer clear of complex jargon and opt for simple, understandable language. This ensures that both seasoned and new developers can grasp your code easily. Keep inline comments under 72 characters for better readability.
- Use clear, descriptive variable and function names to reduce the need for lengthy comments.
- Adhere to the Python PEP 8 style guide for consistent comment formatting and structure.
- Consider refactoring code instead of relying heavily on comments to explain complex logic.
By following these guidelines, your Python code will remain readable, maintainable, and accessible. Well-crafted comments are invaluable, enhancing your code’s quality and understanding.
Commenting Out Code in Integrated Development Environments (IDEs)
As a Python developer, you’ll find that Integrated Development Environments (IDEs) like Visual Studio Code, PyCharm, and Sublime Text offer valuable built-in features for commenting out multiple lines of code. These powerful tools can significantly enhance your coding efficiency and workflow.
Built-in Features of Popular IDEs
Most leading Python IDEs provide seamless ways to comment and uncomment code. In Visual Studio Code and PyCharm, you can use the keyboard shortcut Ctrl + /
(Windows/Linux) or Command + /
(Mac) to toggle comments on the selected lines. Sublime Text users can leverage the same convenient shortcut.
These IDEs also support block commenting and uncommenting, making it easy to disable or re-enable large sections of code quickly. This feature is particularly useful when you need to temporarily disable certain functionalities or experiment with alternative implementations without deleting the original code.
Keyboard Shortcuts for Commenting
- Visual Studio Code and PyCharm:
Ctrl + /
(Windows/Linux) orCommand + /
(Mac) - Sublime Text:
Ctrl + /
(Windows/Linux) orCommand + /
(Mac)
Familiarizing yourself with these keyboard shortcuts can significantly improve your Python development workflow and help you maintain a well-organized and commented codebase.
“Effective commenting is crucial for collaboration and code clarity, providing explanations, aiding in debugging, and organizing code.”
By leveraging the powerful commenting features built into popular Python IDEs, you can streamline your development process, maintain a clean and well-documented codebase, and collaborate more effectively with other developers.
Disabling Blocks of Code Temporarily
Disabling code blocks temporarily is a key debugging and testing technique in Python. It lets developers remove functionality without deleting the code. This makes it simple to revert changes or test different approaches. It’s especially useful in complex projects or when solving codebase issues.
Why Disable Code?
There are several reasons to temporarily disable code blocks in Python:
- Debugging: It helps isolate problems by removing parts of the code.
- Testing: It’s great for testing new features or implementations without changing the code permanently.
- Removing Functionality: It allows you to remove certain functionalities without deleting the code.
How to Safely Comment Out Code
To safely comment out code in Python, you have a few options:
- Use multi-line string comments: Enclose the code in triple quotes (”’ or “””).
- Use consecutive single-line comments: Start each line with a hash symbol (#).
When commenting out nested code, keep the indentation the same. This keeps the code’s structure intact. For big sections, use your IDE’s commenting features or shortcuts.
Always test your code after enabling any commented-out sections. This ensures there are no errors or inconsistencies introduced.
“Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” – Brian Kernighan
Common Mistakes to Avoid
Commenting your Python code is essential for keeping it clear and facilitating teamwork with other developers. Yet, there are a few common errors to avoid in Python code commenting.
Avoiding Over-Commenting
One major mistake is over-commenting. While comments are vital, too many can overwhelm your code. Strive to only add comments where the code isn’t clear or needs extra context.
Keeping Comments Up-to-Date
Another frequent error is neglecting to update comments when the code changes. Outdated comments can mislead other developers. It’s crucial to regularly review and update your Python code maintenance comments.
Mistake | Impact | Solution |
---|---|---|
Over-Commenting | Clutters codebase, reduces readability | Comment only on code that requires additional context |
Forgetting to Update Comments | Leads to misleading information, confuses developers | Review and update comments regularly as code changes |
Avoiding these Python coding errors and keeping your codebase clean and well-commented ensures your projects are easy to grasp, maintain, and work on with others.
Example Scenarios for Commenting in Python
Comments are vital in Python, boosting code readability and upkeep. Let’s delve into scenarios where comments greatly enhance your Python code’s quality.
Comments in Function Definitions
Comments in function definitions are a common use. Docstrings offer a detailed overview of the function’s purpose, parameters, and return values. This clarity aids in understanding the code’s intent, simplifying maintenance and collaboration.
Providing Context in Complex Logic
Python’s capabilities enable the creation of complex algorithms. In these instances, well-placed comments elucidate the logic behind your choices. This contextual insight is crucial for complex code or mathematical operations.
For instance, comments can detail data processing steps or the formulas used in calculations. They also shed light on API or library integrations within your codebase.
Through these comments, your Python code remains clear and manageable, even as projects evolve.
The aim of commenting in Python is to offer useful information without clutter. Aim for clarity, brevity, and relevance in your comments. This ensures your code and documentation are well-understood.
Conclusion
In the realm of Python programming, mastering the art of commenting is vital. It significantly boosts code readability and maintainability. This article has delved into various commenting techniques and best practices in Python. We’ve covered everything from single-line comments to multi-line docstrings.
Summarizing Key Takeaways
Our discussion highlighted the importance of selecting the right comment type for each task. It also emphasized the role of Integrated Development Environments (IDEs) in streamlining the commenting process. Additionally, we stressed the need for keeping comments organized and current. By adhering to these principles, Python programmers can craft code that is not only functional but also transparent to others and their future selves.
Encouraging Good Commenting Practices
As you advance in your Python coding endeavors, remember that commenting is a cornerstone of Python coding excellence and programmer skills. Dedicate time to crafting clear, concise, and pertinent comments. This ensures your code remains well-documented and maintainable. Engaging in regular code reviews can further enhance your commenting skills. It will make you a more proficient and valuable Python programmer.
FAQ
How can I comment out multiple lines in Python?
Python doesn’t have a direct syntax for multi-line comments. However, you can use consecutive single-line comments or create multi-line string comments. This approach allows you to comment out multiple lines effectively.
What are comments in Python?
Comments in Python are texts ignored by the interpreter. They serve to explain the code, provide documentation, and enhance readability.
Why is it important to comment code in Python?
Commenting code is essential for maintenance, debugging, and collaboration. It aids in understanding the code’s purpose, usage, and implementation details.
When should I use single-line comments vs. multi-line comments in Python?
Single-line comments are best for brief explanations or inline notes. For longer descriptions or function documentation, use multi-line comments. They are also useful for commenting out large code blocks.
How do I use the hash symbol (#) to create multi-line comments in Python?
To create multi-line comments with the hash symbol, prefix each line with #. This method is simple but can be cumbersome for extensive code blocks.
How can I use multi-line strings as comments in Python?
Python allows using triple quotes for multi-line strings as comments. This method is beneficial for commenting out large sections of code without adding a # to each line.
What are some best practices for commenting in Python?
Comments should be concise, clear, and relevant. Update them when the code changes. Use simple language and avoid jargon. Follow PEP 8 for consistency. Refactor code instead of over-commenting, and regularly remove outdated comments.
How can I use integrated development environments (IDEs) to comment out multiple lines of code in Python?
IDEs like Visual Studio Code, PyCharm, and Sublime Text offer features for commenting out lines. Use the keyboard shortcut Ctrl + / (Windows/Linux) or Command + / (Mac) to toggle comments on selected lines.
When and why would I want to temporarily disable code blocks in Python?
Disabling code blocks is useful for debugging, testing, or removing functionality temporarily. Use multi-line string comments or consecutive single-line comments to comment out code safely.
What are some common mistakes to avoid when commenting in Python?
Avoid over-commenting obvious code and neglecting to update comments when the code changes. Also, be cautious with inline comments that may disrupt code formatting.
In what scenarios would I use comments in my Python code?
Use comments to describe function purposes, parameters, and return values. They are also useful for explaining complex logic, providing context for data processing, or detailing the use of external APIs and libraries.